Wall Slide, Jump and Grab Mechanics With Unreal Engine Blueprints
Imagine a nimble character, quick on their feet, leaping from wall to wall with ease, clinging to ledges, and sliding down surfaces in a thrilling dance of agility and skill. In this article, I’ll take you through the process of developing these gameplay mechanics in Unreal Engine using both Blueprints and C++.
Now, let’s dive into the first steps of bringing this character to life, starting with the fundamentals of wall sliding and wall jumping.
Wall Slide
First up is the wall slide, letting our character gracefully descend along a wall rather than plummeting straight down. To bring this to life in Unreal Engine, it’s implemented in two parts.
First, with WallSlideCapsuleSweep
, which checks for a nearby wall by casting a capsule trace forward from the character’s position, returning true if there’s a detection of surfaces in front of it.
Second, in the tick, the WallSlide
function is executed, checking the capsule sweep to detect a wall and confirming that the character’s Vertical velocity is negative (moving downwards). Then, the wall slide is activated by gradually reducing this fall speed using the interp function VInterpConstantTo
for a controlled, smooth descent.
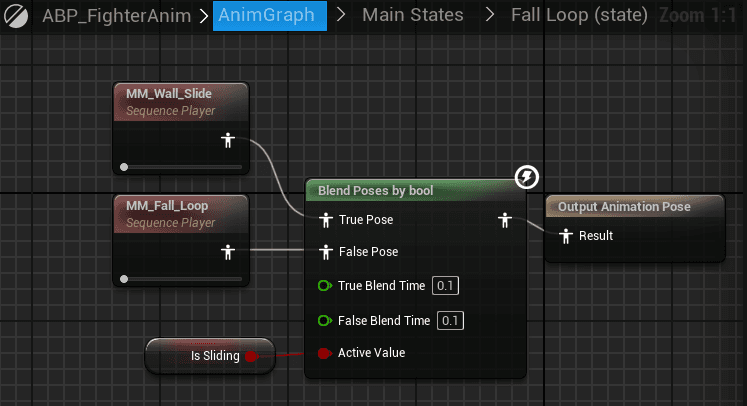
Now, all it takes to visually represent this slide is a bit of animation and VFX magic. By checking if wall sliding is active (bWallSliding
), we can easily apply an animation blend on the falling loop, smoothly transitioning into a wall slide animation that matches the pose.
Check the code in Blueprints
Check the code in C++
Wall Jump
Next up is the wall jump, a dynamic move that lets our character kick off walls and launch into the air, opening up new ways to navigate the environment.
For this, it's a simple trick. On your Jump Action, we check if the player is sliding, and then we zero out the velocity and launch the player in the opposite direction using a repel force
variable.
Check the code in Blueprints
Check the code in C++
Wall Grab
Finally, we have the wall grab a mechanic that lets our character grab onto ledges, providing players with a moment to catch their breath, strategize, or set up their next jump.
I started by implementing two capsule sweeps. The first one, WallGrabDetectionCapsuleSweep
, is used to detect if the character is in range to grab a ledge by casting a capsule trace in front of the character. If a valid ledge is found, it records the impact point and determines whether the ledge can be auto-grabbed. The second capsule sweep, WallGrabHandCapsuleSweep
, ensures the character’s hands are in the correct position to grab the ledge, adding an extra level of precision.
Once a ledge is detected, the AttachToLedge
function is called to handle the actual attachment. This function disables movement, plays the grab animation, and sets the character into a flying mode, effectively locking them in place on the ledge. Conversely, the DetachFromLedge
function is responsible for releasing the character, re-enabling movement, and restoring collision, allowing the character to drop or jump away from the ledge.
To ensure the character is precisely positioned on the ledge, the FixCharacterToLedge
function is used. It adjusts the character’s location and rotation, aligning them perfectly with the ledge and ensuring a visually accurate attachment. This function is crucial for avoiding clipping issues and making the interaction feel polished.
In the tick function, the WallGrab
function continuously checks for conditions that would allow a wall grab to occur. By using a boolean (bAutoGrabbing
), we can make the wall grab mechanic automatic, allowing the character to hang onto ledges without extra player input when conditions are met. Additionally, the DetachFromLedge
function is linked to the jump action, enabling the character to detach from the ledge and transition smoothly into a jump when the jump input is triggered.
Check the code in Blueprints
Check the code in C++